Timed Out After Waiting 30000ms
How to resolve Puppeteer timeout errors
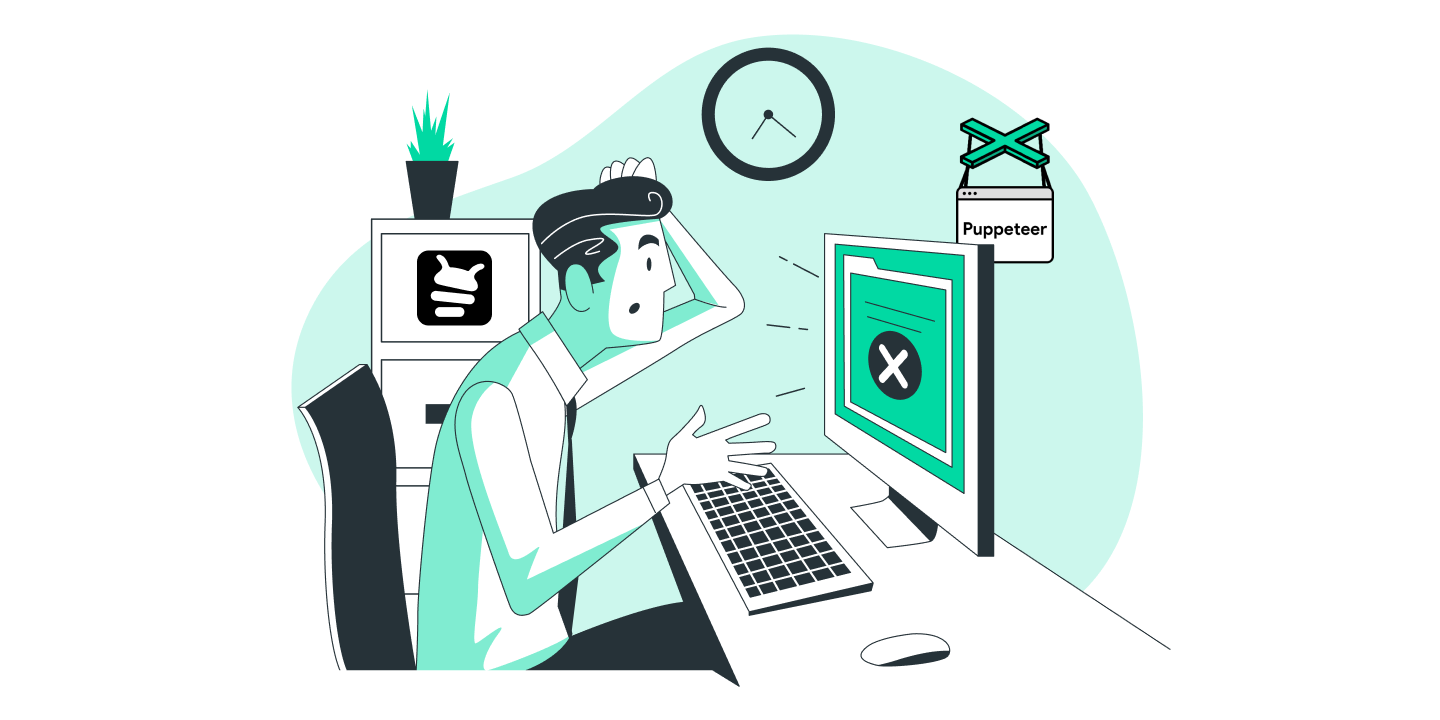
The error Timed Out After Waiting 30000ms occurs when a Puppeteer script takes more than 30 seconds to perform the requested action. This timeout is a built-in safety feature designed to prevent scripts from running indefinitely.
How to Fix the Error
To resolve this issue, you can increase the timeout duration for the specific action. For example, if you are waiting for a page to load, increase the timeout for the page.waitForNavigation
function:
await page.waitForNavigation({ timeout: 60000 });
In this case, the timeout is extended to 60 seconds.
Alternatively, if you are waiting for a specific element to appear, you can adjust the timeout for the page.waitForSelector
function:
await page.waitForSelector('selector', { timeout: 60000 });
Most Puppeteer functions include a timeout option, allowing you to customize the waiting period for various actions.
Still Encountering Issues?
If increasing the timeout does not solve the problem, consider saving the page content to a file for further analysis. You can also use a visual testing tool like Buglesstack to debug your automation visually.
import axios from 'axios';
import puppeteer from 'puppeteer';
const browser = await puppeteer.launch();
const page = await browser.newPage();
try {
await page.goto('https://www.google.com/search?q=puppeteer');
await page.waitForSelector('[name="q"]');
await page.close();
await browser.close();
}
catch (error) {
// Prepare buglesstack data
const buglesstackData = {
url: page.url(),
screenshot: await page.screenshot({ encoding: 'base64' }),
html: await page.content(),
metadata: {},
message: error.message,
stack: error.stack
};
// Get your access token from https://app.buglesstack.com
const ACCESS_TOKEN = 'HERE_YOUR_ACCESS_TOKEN';
// Send error to buglesstack
await axios.post('https://app.buglesstack.com/api/v1/crashes', buglesstackData, {
headers: {
Authorization: `Bearer ${ACCESS_TOKEN}`,
'Content-Type': 'application/json'
}
});
// Re-throw the error to propagate it
throw error;
}
In this example, error details are captured using Buglesstack, which allows you to further analyze the issue while preserving the error chain.
By adjusting timeouts and employing debugging tools, you can effectively address Puppeteer timeout errors and improve the stability of your scripts.