No usable sandbox! Error in Puppeteer
Learn how to fix the 'No usable sandbox' error when running Puppeteer in Docker or restricted environments
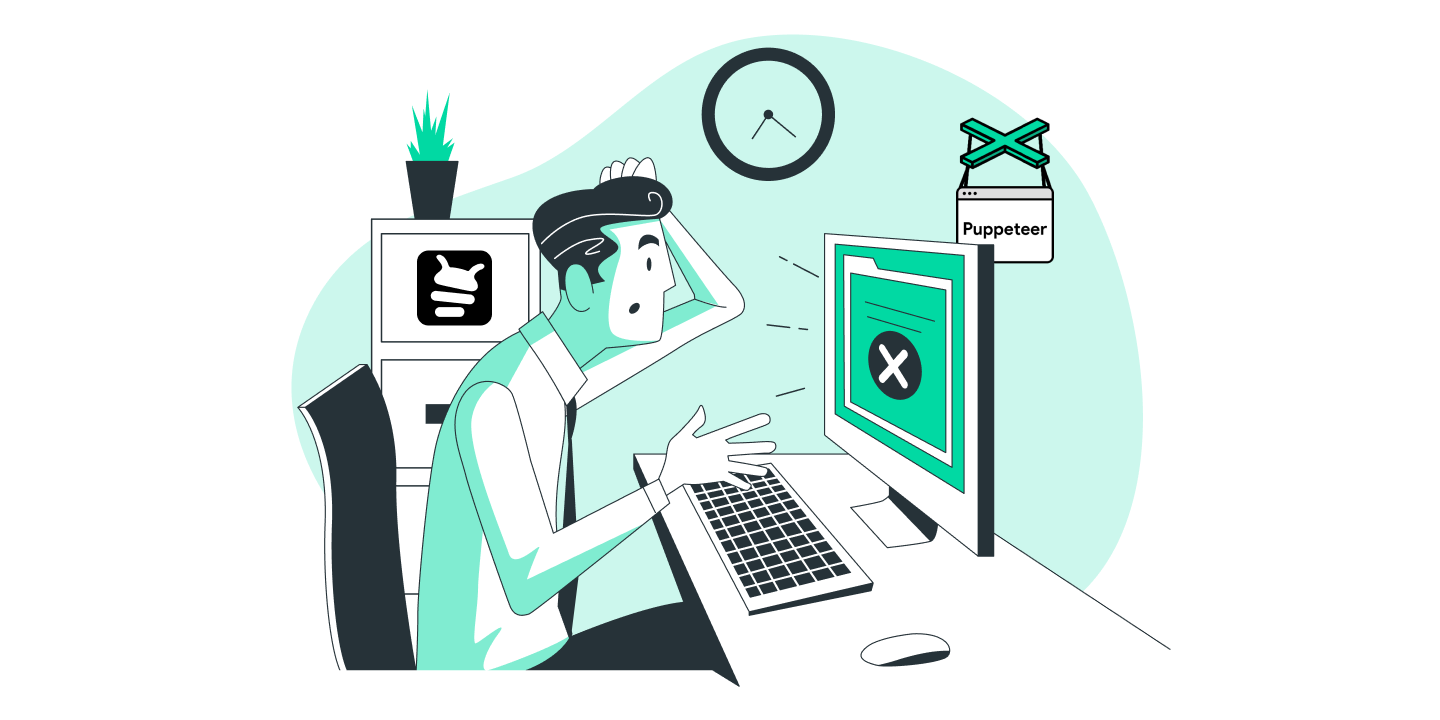
This error typically occurs when running Puppeteer in Docker containers or environments with restricted permissions. The Chrome sandbox is a security feature that needs proper configuration to work.
Common Causes
- Docker container restrictions: Running in a container without proper sandbox configuration
- Root user execution: Running Chrome as root user
- Missing system dependencies: Required system libraries are not installed
- Incorrect Puppeteer launch options: Sandbox settings not properly configured
Solutions
1. Disable the sandbox (Not recommended for production)
const browser = await puppeteer.launch({
args: ['--no-sandbox', '--disable-setuid-sandbox']
});
2. Configure Docker properly
Add these capabilities to your Docker container:
FROM node:18
# Install latest chrome dev package and fonts to support major charsets
RUN apt-get update \
&& apt-get install -y wget gnupg \
&& wget -q -O - https://dl-ssl.google.com/linux/linux_signing_key.pub | apt-key add - \
&& sh -c 'echo "deb [arch=amd64] http://dl.google.com/linux/chrome/deb/ stable main" >> /etc/apt/sources.list.d/google.list' \
&& apt-get update \
&& apt-get install -y google-chrome-stable fonts-ipafont-gothic fonts-wqy-zenhei fonts-thai-tlwg fonts-kacst fonts-freefont-ttf libxss1 \
--no-install-recommends \
&& rm -rf /var/lib/apt/lists/*
# Create a non-root user
RUN groupadd -r pptruser && useradd -r -g pptruser -G audio,video pptruser \
&& mkdir -p /home/pptruser/Downloads \
&& chown -R pptruser:pptruser /home/pptruser
# Run everything after as non-privileged user
USER pptruser
3. Install required system dependencies
For Ubuntu/Debian:
sudo apt-get update
sudo apt-get install -y \
ca-certificates \
fonts-liberation \
libasound2 \
libatk-bridge2.0-0 \
libatk1.0-0 \
libc6 \
libcairo2 \
libcups2 \
libdbus-1-3 \
libexpat1 \
libfontconfig1 \
libgbm1 \
libgcc1 \
libglib2.0-0 \
libgtk-3-0 \
libnspr4 \
libnss3 \
libpango-1.0-0 \
libpangocairo-1.0-0 \
libstdc++6 \
libx11-6 \
libx11-xcb1 \
libxcb1 \
libxcomposite1 \
libxcursor1 \
libxdamage1 \
libxext6 \
libxfixes3 \
libxi6 \
libxrandr2 \
libxrender1 \
libxss1 \
libxtst6 \
lsb-release \
wget \
xdg-utils
4. Use a non-root user
If running as root, switch to a non-root user:
sudo useradd -m -s /bin/bash puppeteer
sudo chown -R puppeteer:puppeteer /path/to/your/app
sudo -u puppeteer node your-script.js
Still Encountering Issues?
If you’re still having trouble with the sandbox configuration, you can use a visual testing tool like Buglesstack to debug your automation setup and verify browser configurations.
try {
await page.goto('https://www.google.com/search?q=puppeteer');
await page.waitForSelector('[name="q"]');
}
catch (error) {
// Prepare buglesstack data
const buglesstackData = {
url: page.url(),
screenshot: await page.screenshot({ encoding: 'base64' }),
html: await page.content(),
metadata: {},
message: error.message,
stack: error.stack
};
// Get your access token from https://app.buglesstack.com
const ACCESS_TOKEN = 'HERE_YOUR_ACCESS_TOKEN';
// Send error to buglesstack
await axios.post('https://app.buglesstack.com/api/v1/crashes', buglesstackData, {
headers: {
Authorization: `Bearer ${ACCESS_TOKEN}`,
'Content-Type': 'application/json'
}
});
// Re-throw the error to propagate it
throw error;
}
By properly configuring the sandbox and system permissions, you can resolve the “No usable sandbox” error and run your automation securely.