Could not find Chrome (ver. 133.0.6943.98)
Learn how to fix the 'Could not find Chrome' error when Puppeteer cannot find a specific Chrome version
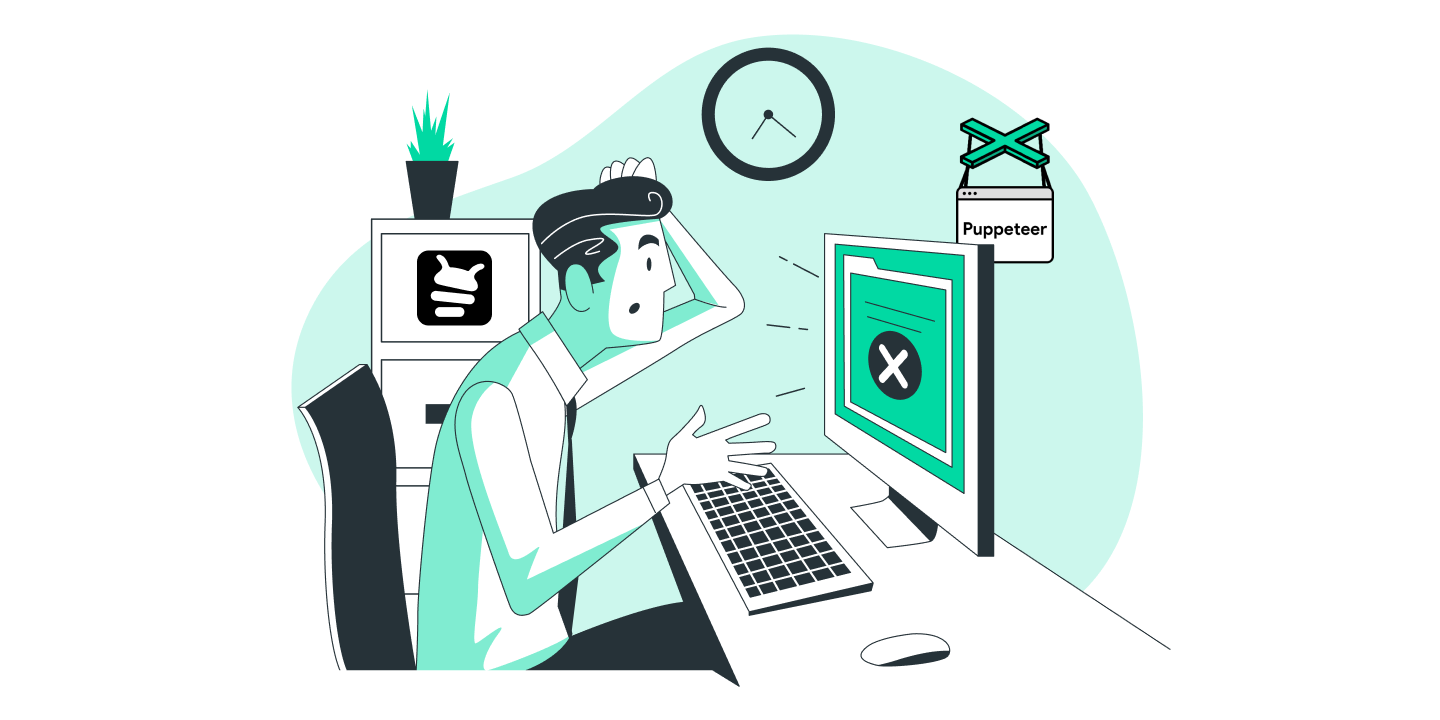
This error occurs when Puppeteer is looking for a specific version of Chrome that isn’t installed on your system. This is common when using puppeteer-core
or when there’s a version mismatch.
Common Causes
- Version mismatch: Installed Chrome version doesn’t match Puppeteer’s requirements
- Using puppeteer-core:
puppeteer-core
doesn’t download browsers automatically - Corrupted installation: Chrome installation is incomplete or damaged
- Incorrect version specification: Wrong version number in configuration
Solutions
1. Use puppeteer instead of puppeteer-core
Switch to puppeteer
to automatically download the correct Chrome version:
// Remove puppeteer-core
// npm uninstall puppeteer-core
// Install puppeteer
// npm install puppeteer
const puppeteer = require('puppeteer');
const browser = await puppeteer.launch();
2. Install the specific Chrome version
For Ubuntu/Debian:
# Download specific version
wget https://dl.google.com/linux/direct/google-chrome-stable_133.0.6943.98-1_amd64.deb
# Install
sudo dpkg -i google-chrome-stable_133.0.6943.98-1_amd64.deb
sudo apt-get install -f
For macOS:
# Using Homebrew
brew install --cask google-chrome --version=133.0.6943.98
3. Specify a compatible Chrome version
If you can’t install the exact version, use a compatible one:
const browser = await puppeteer.launch({
executablePath: '/path/to/chrome',
product: 'chrome',
// Use a version that's installed on your system
args: ['--version=133.0.6943.98']
});
4. Clear Puppeteer cache and reinstall
# Remove Puppeteer cache
rm -rf ~/.cache/puppeteer
# Reinstall Puppeteer
npm uninstall puppeteer
npm install puppeteer
5. Check Chrome version
Verify your installed Chrome version:
# For Linux
google-chrome --version
# For macOS
/Applications/Google\ Chrome.app/Contents/MacOS/Google\ Chrome --version
# For Windows
"C:\Program Files\Google\Chrome\Application\chrome.exe" --version
Still Encountering Issues?
If you’re still having trouble with Chrome version compatibility, you can use a visual testing tool like Buglesstack to debug your automation setup and verify browser configurations.
// .. assuming you already have your Puppeteer browser and page initialized
try {
await page.goto('https://www.google.com/search?q=puppeteer');
await page.waitForSelector('[name="q"]');
}
catch (error) {
// Prepare buglesstack data
const buglesstackData = {
url: page.url(),
screenshot: await page.screenshot({ encoding: 'base64' }),
html: await page.content(),
metadata: {},
message: error.message,
stack: error.stack
};
// Get your access token from https://app.buglesstack.com
const ACCESS_TOKEN = 'HERE_YOUR_ACCESS_TOKEN';
// Send error to buglesstack
await axios.post('https://app.buglesstack.com/api/v1/crashes', buglesstackData, {
headers: {
Authorization: `Bearer ${ACCESS_TOKEN}`,
'Content-Type': 'application/json'
}
});
// Re-throw the error to propagate it
throw error;
}
By ensuring proper Chrome version installation and configuration, you can resolve the “Could not find Chrome” error and get your automation running smoothly.